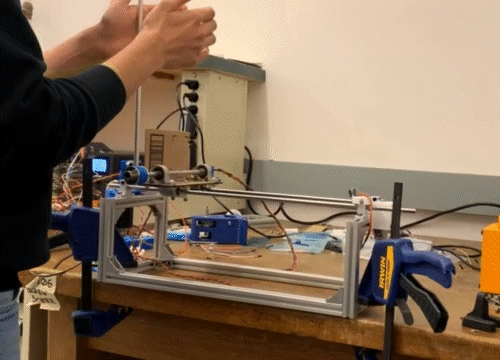
Inverted Pendulum
Designed a real-time control system to evaluate the performance of a Byzantine Fault Tolerant key-value store.
Learn moreThis project extends FreeRTOS by implementing two major features: an Earliest Deadline First (EDF) scheduling algorithm and Stack Resource Policy (SRP) mechanisms and provides an API for user access that follows FreeRTOS conventions. FreeRTOS is a popular open-source real-time operating system (RTOS) designed for embedded systems, enabling precise task scheduling and resource management. These enhancements improve task prioritization and resource management in real-time systems. The project was developed on a Raspberry Pi Pico using the C programming language. I completed this project as part of CPSC 538G Real-Time Systems at UBC.
EDF (Earliest Deadline First): A dynamic scheduling algorithm that prioritizes tasks based on their deadlines. The task with the earliest deadline is executed first, ensuring time-critical operations are performed within their constraints.
SRP (Stack Resource Policy): A protocol to manage resource access in priority-driven systems. It prevents priority inversion and deadlocks by assigning a system ceiling to resources and ensuring tasks adhere to these ceilings.
With my implementation, users can call the xTaskCreateEDF
function to create tasks with periodic execution. It calculates activation times and absolute deadlines dynamically for each task. Admission control ensures that tasks are only admitted if system utilization allows.
The project integrates Stack Resource Policy (SRP) mechanisms into FreeRTOS to manage resource access in systems with priority inheritance requirements. This ensures efficient and deterministic resource sharing.
SRP_GetCurrentCeiling:
Retrieves the current system ceiling.xSRPCreateBinary:
Creates semaphores with SRP properties.xSRPSemaphoreTake:
Acquires an SRP semaphore.xSRPSemaphoreGive:
Releases an SRP semaphore.The SRP mechanisms prevent priority inversion by enforcing system ceilings and simplify real-time system design.
The enhancements were validated using demo workloads with the pico connected to various LEDs to visually demonstrate different tasks running. Tests verified the EDF scheduler's task execution order, deadline enforcement, and system utilization. For SRP, tasks were tested for deterministic resource sharing and priority inversion prevention. Initial testing involved simply stepping through the code to ensure correctness.
Challenges:
Lessons Learned:
Designed a real-time control system to evaluate the performance of a Byzantine Fault Tolerant key-value store.
Learn moreTrained a rover to drive on a simulated road, detecting license plates and avoiding obstacles.
Learn more